Hi all, it has certainly been a while, even though the only comments I get are from bizarre spam bots. As always there is a TLDR for you down below. So lets get into it.
Have you ever been in a situation where you make a lovely custom dropdown menu for someone and you do not know how to close the dropdown when you or they click away?
Well this is where a directive comes in and we even get to use fancy Rxjs in the mix.
Directives are defined as classes that can add new behavior to the elements in the template or modify existing behavior.
First off you will need to create your directive would recommend using the Angular CLI tool on this one.

// Angular generate new service
ng g s servicename
[Pro tip]: If you are unsure where the CLI tool will place your directive you can use the extension –dry-run.
We will be using RXjs in this directive to listen to the clicking of the element outside the assigned element
TLDR here is the Directive code to handle the clicking outside directive. Be sure to declare the Directive in your module that you are using said directive.
import { DOCUMENT } from "@angular/common";
import { AfterViewInit, Directive, ElementRef, EventEmitter, Inject, OnDestroy, Output } from "@angular/core";
import { filter, fromEvent, Subscription } from "rxjs";
@Directive({
selector: '[appClickOutside]',
})
export class ClickOutsideDirective implements AfterViewInit, OnDestroy {
@Output() appClickOutside = new EventEmitter<void>();
documentClickSubscription: Subscription | undefined;
constructor(
private element: ElementRef,
@Inject(DOCUMENT) private document: Document,
) { }
ngAfterViewInit(): void {
// you are listening for a click anywhere on the document
this.documentClickSubscription = fromEvent(this.document, 'click')
.pipe(
filter((event) => {
// the Rxjs filter will return when a click is not within the directive element
return !this.isInside(event.target as HTMLElement);
}),
)
.subscribe(() => {
// upon the case of element clicked outside the subscription will be emitted
this.appClickOutside.emit();
});
}
ngOnDestroy(): void {
this.documentClickSubscription?.unsubscribe();
}
isInside(elementToCheck: HTMLElement): boolean {
return (
elementToCheck === this.element.nativeElement ||
this.element.nativeElement.contains(elementToCheck)
);
}
}
How does this directive work?
The finer detail is that the subscription is being used to subscribe to specifically where the user is clicking on the document. The pipe contains a filter which checks if the user has clicked off the element or on the element, this is where we subscribe to said subscription to when the event has been clicked outside the element. The emitter will emit the appClickOutside emitter.
I don’t care how it works, what else must I do to get this setup in my project!
Assuring that you have created your directive and have declared it in your module next you will need to update the view. On the view of your component you will need to add the appClickOutside directive to the element that wraps around your custom dropdown menu, as you can see on line 2 I have a dropdown wrapper class to ensure that I have the area of the dropdown covered so you may need to specify the height or width depending on your layout.
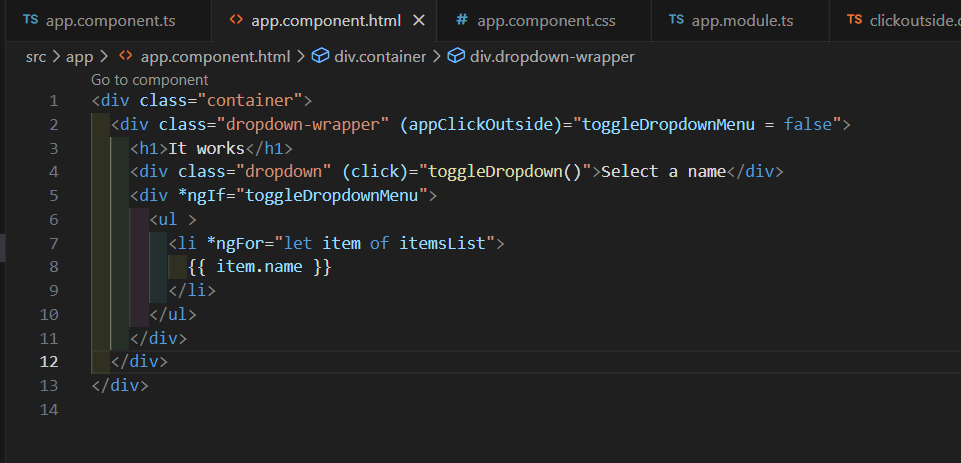
If the click off is not quite working, I’d advice to take look at your styles and element wrapper.
Ya that is about it. Feel free to take a look at my working sample on github if you are facing any challenges