I wanted to make a whole story on this, but i’d rather try make this document as helpful and to the point as possible, hope this helps you setup jest in your Angular project.
import jest setup that is found in the jest-preset-angular.
This will be in your test.ts file
// add to test.ts file
import 'jest-preset-angular/setup-jest';
Add the following jest packages the the package.json
// package.json file
// update your test script to
"test": "jest",
// Packages to add to devDependencies
"jest": "^27.3.1",
"jest-preset-angular": "^10.0.1"
After adding the jest packages be sure to run npm install, you can also remove the karma packages to keep things tidy.
In the project’s tsconfig.json add the following to your compilerOptions object:
// add the following in your tsconfig.json
...,
"angularCompilerOptions": {
"fullTemplateTypeCheck": true,
"strictInjectionParameters": true
}
Run the following command, this will help transcript jest to typescript seeing that jest is JavaScript and will need to work with type script.
npm i ts-jest
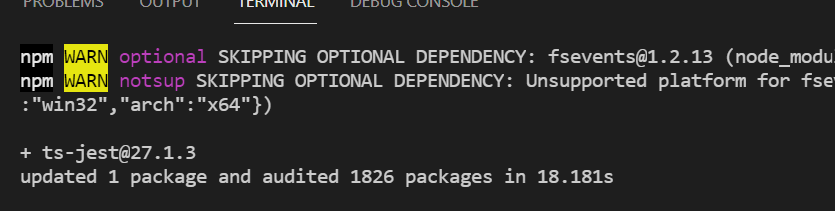
Create a jest.config.js file in the base of your project ‘src/jest.config.js’
const { pathsToModuleNameMapper } = require('ts-jest');
const { compilerOptions } = require('./tsconfig.json');
module.exports = {
preset: "jest-preset-angular",
setupFilesAfterEnv: ["<rootDir>/src/test.ts"],
testMatch: ["**/+(*.)+(spec).+(ts)"],
moduleNameMapper: pathsToModuleNameMapper(compilerOptions.paths || {}, {prefix: "<rootDir>/"})
}
I think that that should sort you out. With regards to the different versions of Angular and Jest, and its angular preset packages, they seem to be inline with the angular version number, like my project is angular 11 and I have another using 12 and the preset 10 and up seems to cover it. I also have used it on an Angular 9 project and the jest-preset-angular version was 9.0.4
EXTRA SETUP NOTE FOR JEST
I had to added the Jest to the types in the tsconfig.spec.json, so that my unit test files have access to jest.
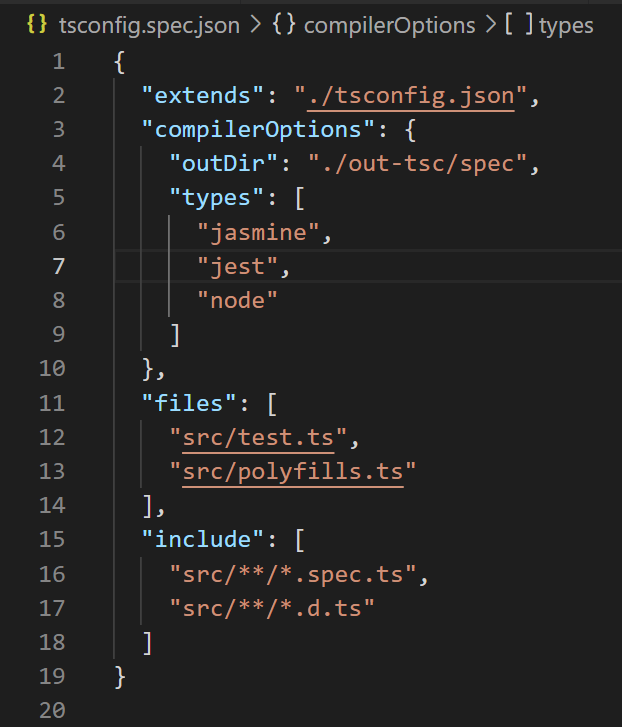
Sample project with Jest setup already:
sample-project