I’m gonna cut to the chase, so no story time here.
First off here is the documentation for the @ngbootrap Modal !
To install and setup first
run this command line to get access to the @ng-bootstrap/ng-bootstrap node modules.
npm i @ng-bootstrap/ng-bootstrap
Once you have installed the files make a new component for the Modal itself
You would need to import the NgbModal with the line below.
// import into modal.component.ts file
import { NgbModal } from '@ng-bootstrap/ng-bootstrap';
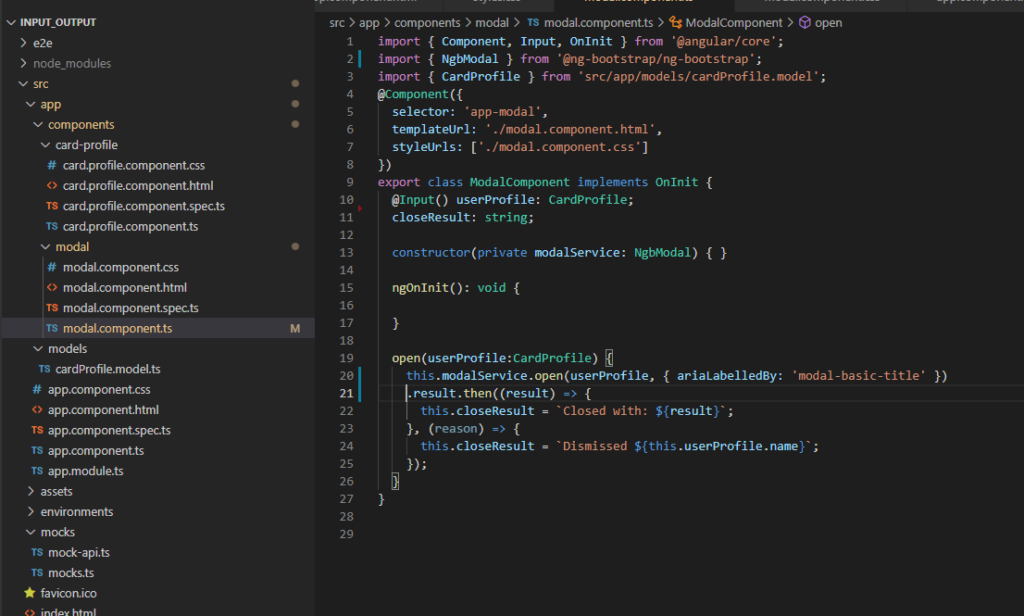
For this sample I am using the NgbModal. If you take a look at the documentation it has a list of APIs associated with the NgbModal and we are going to use some of the basic samples to get this up and running.
For the modal.component.html view what is nice is that you can use conditional rendering if you want to hide some of the details. or comment it out…I was having issues toggling and where to hide and show these details and I think this is the simplest way to manage with my specific demo project.
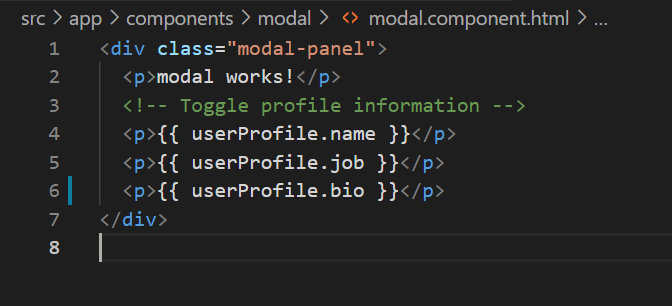
I have a working Angular 9 project that I have setup to emit a value of a userProfile component that contains the details of Name, Job and bio.
I was thinking of going through the code line by line but you’ll need to pass data to the component and through the emitter the function will emit the value to the parent component and now to create the component instance. So in the view you can see I’m using the event emitter value from the card profile component, so
this ‘(selectedProfile)= parentFunction(userProfile)” is going to help us create this component instance of the bootstrap modal in the main app component .
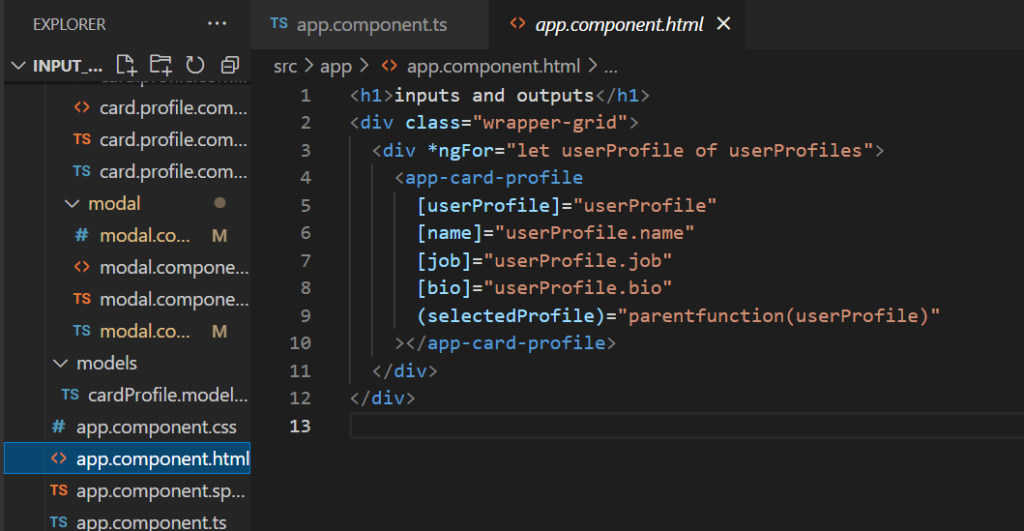
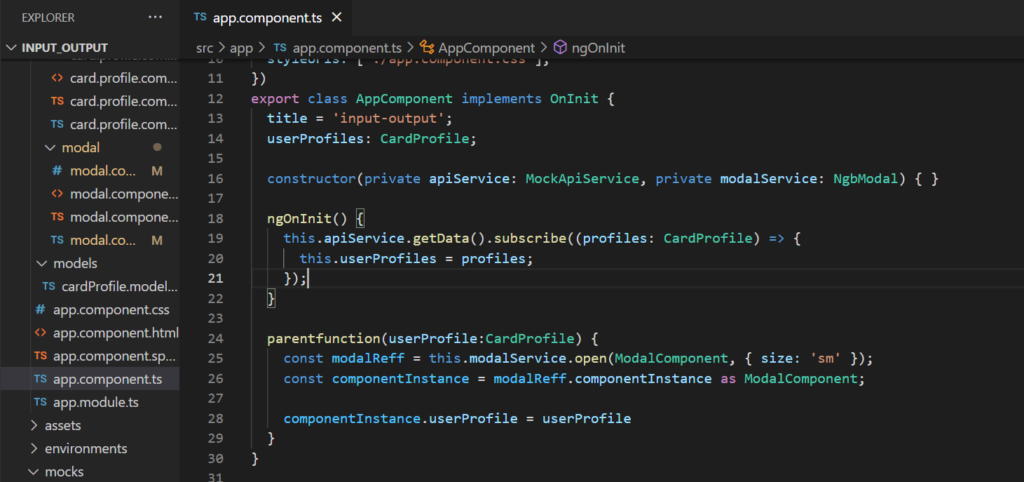
parentfunction(userProfile:CardProfile) {
const modalReff = this.modalService.open(ModalComponent, { size: 'sm' });
const componentInstance = modalReff.componentInstance as ModalComponent;
componentInstance.userProfile = userProfile
}
The above is where the magic happens, upon clicking the parentFunction it’ll create a const modalReff. from there you can reference the componentInstance and should have access to the ModalComponent’s inputs and variables.
Granted its a bit of setup work but this is how one would use a component instance in a project
If you have any issues you can check out the working sample project right here
this carries examples of inputs and outputs