If you want the sample main steps scroll to the “TO THE POINT” part of this article thanks. If you all good keep reading.
So this lock out isolation thing has been quite challenging for everyone and I feel your pain. I was working on an angular portfolio…but its been mainly css when trying to cater for mobile view and tablet view…so I want to take a break from the css and get into interacting with an API
If you don’t know what an API is…this wont cover the fundamentals sadly, it’ll be a working example of an api request. As for now we are Focusing on Angular working with APIs using services.
There are many free open API sites the one i came across apifootball.com where upon registering they’ll provide you with an API key (you’ll need to keep this safe and secure) From my experience most of the free apis require a registration.
We have an api and an api key, now you want to check and see if we can make a call of some sort and log a response. A great API tool is postman. Basically setup the API call to your liking and it allows you to see and track the response and what not, look its great.
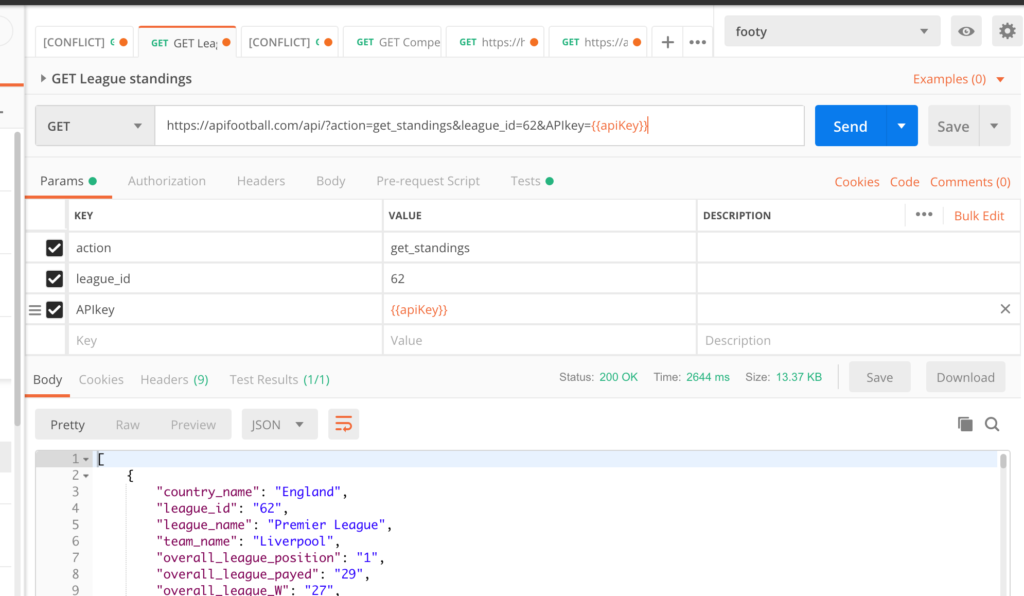
ok so that is the long of it. Now i’m going to try to keep it as short as possible. Yes that does say “overal_league_payed” learned that the hard way.
TO THE POINT HOW TO SETUP TO HIT AN API
Using the angular cli we can just enter the following command in the terminal
// short hand
ng g s serviceName
// full on
ng generate service serviceName
The command will generate a service for you in your solution. Basic boiler plate.
Right now you know your API is working. Let’s make that service in our angular app give that API a call and see if we can get their response.
We need RXJS thats right that beast that does magic with Observables (high level comparison of observables vs promises.).
npm i rxjs
Make sure that you are using HttpClient for the http calls.
I normally use the below structure for api calls. Adding variable for base urls and other things that are constant like my apiKey this is just personal preference.
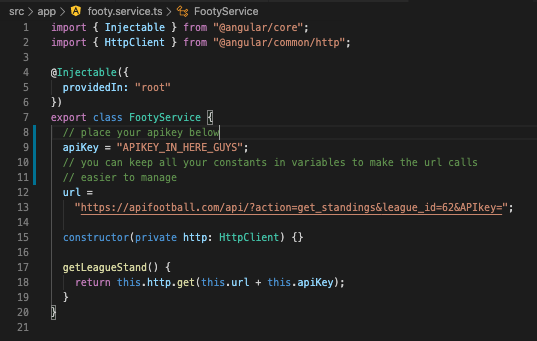
I was advised that when it comes to services, make sure they only deliver the data keep that part clean. Now moving along to the app.component we’ll need to create a function that hits that service and the getLeagueStand and subscribe to the response and then extract the data you want. Make sure you return the url.
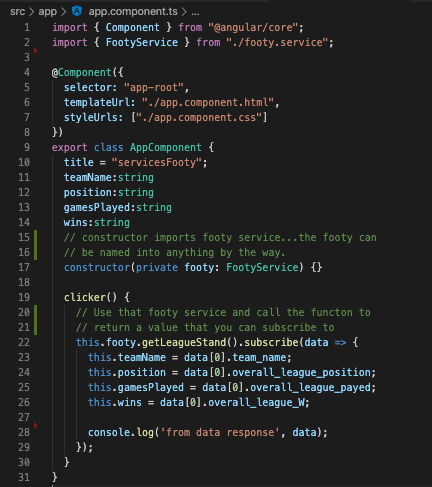
And ALSO do not forget to import your service and the httpClient module to your app.module file like so…I can’t believe I forgot to add this guy.
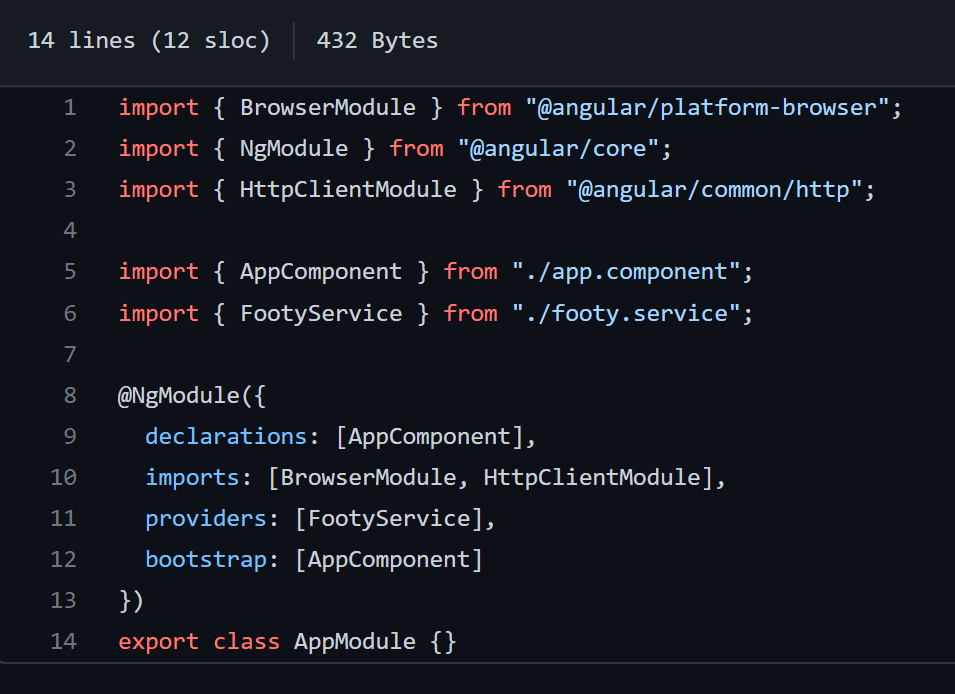
As you can see in the code above we subscribing to the data and then setting out its values to variables. Here is a repo to a working sample. You’ll need to add your own apikey though.